Hadoop框架:HDFS讀寫機制與API詳解
阿新 • • 發佈:2020-09-30
本文原始碼:[GitHub·點這裡](https://github.com/cicadasmile/big-data-parent) || [GitEE·點這裡](https://gitee.com/cicadasmile/big-data-parent)
# 一、讀寫機制
## 1、資料寫入
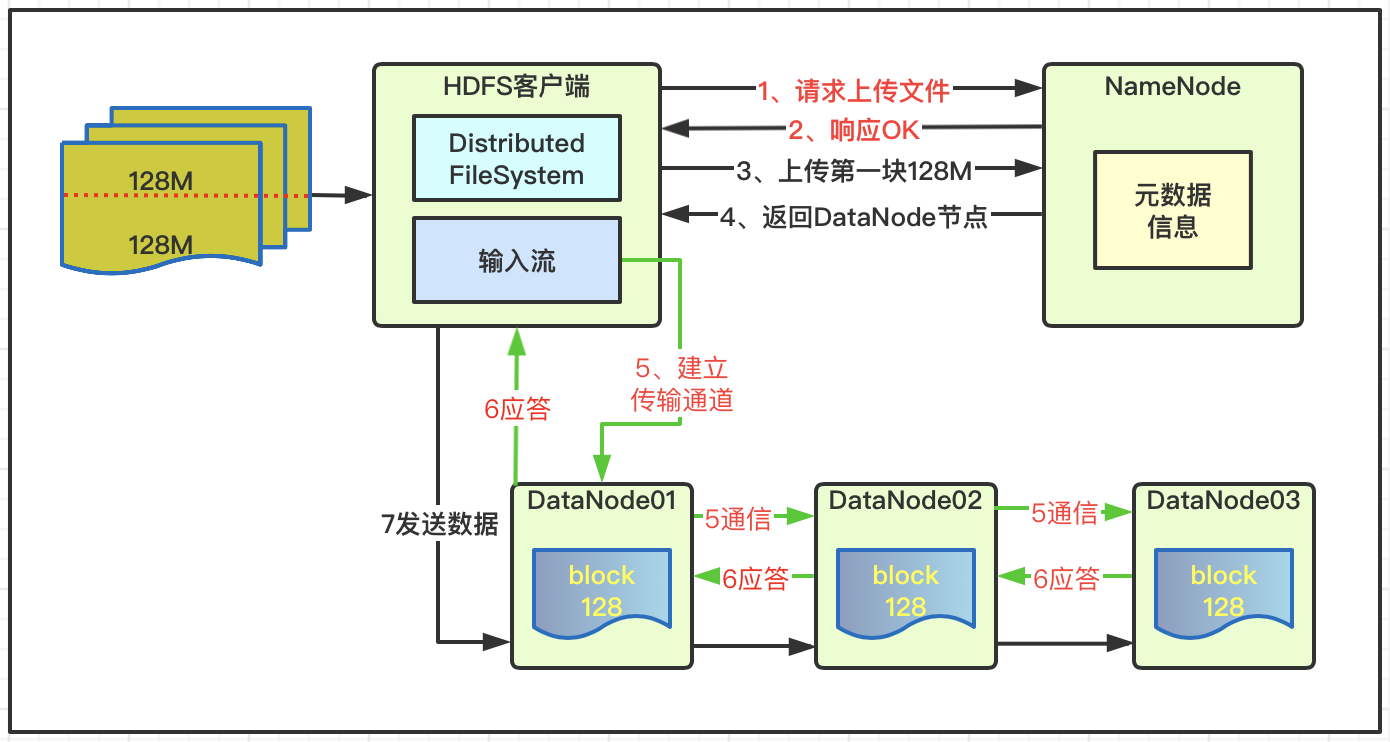
- 客戶端訪問NameNode請求上傳檔案;
- NameNode檢查目標檔案和目錄是否已經存在;
- NameNode響應客戶端是否可以上傳;
- 客戶端請求NameNode檔案塊Block01上傳服務位置;
- NameNode響應返回3個DataNode節點;
- 客戶端通過輸入流建立DataNode01傳輸通道;
- DataNode01呼叫DataNode02,DataNode02呼叫DataNode03,通訊管道建立完成;
- DataNode01、DataNode02、DataNode03逐級應答客戶端。
- 客戶端向DataNode01上傳第一個檔案塊Block;
- DataNode01接收後傳給DataNode02,DataNode02傳給DataNode03;
- Block01傳輸完成之後,客戶端再次請求NameNode上傳第二個檔案塊;
## 2、資料讀取
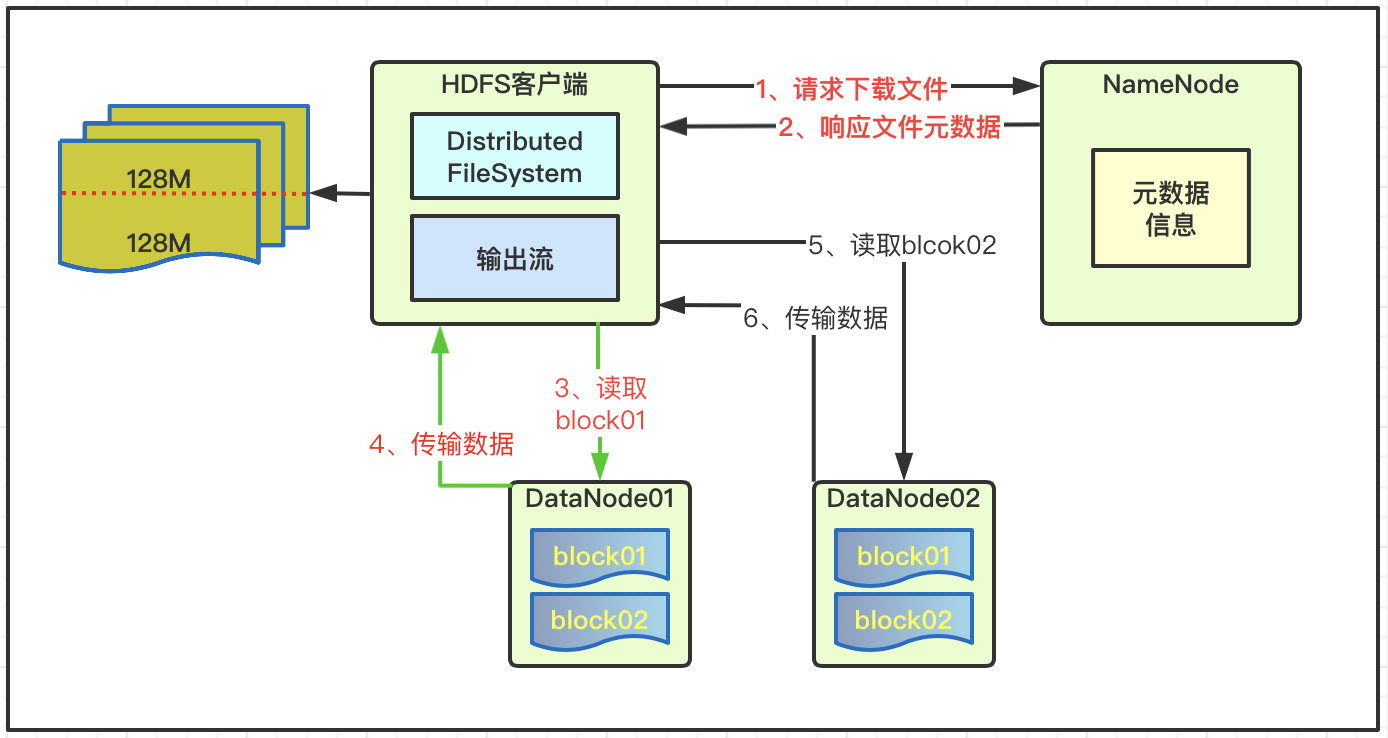
- 客戶端通過向NameNode請求下載檔案;
- NameNode查詢獲取檔案元資料並返回;
- 客戶端通過元資料資訊獲取檔案DataNode地址;
- 就近原則選擇一臺DataNode伺服器,請求讀取資料;
- DataNode傳輸資料返回給客戶端;
- 客戶端以本地處理目標檔案;
# 二、基礎API案例
## 1、基礎演示介面
```java
public interface HdfsFileService {
// 建立資料夾
void mkdirs(String path) throws Exception ;
// 檔案判斷
void isFile(String path) throws Exception ;
// 修改檔名
void reName(String oldFile, String newFile) throws Exception ;
// 檔案詳情
void fileDetail(String path) throws Exception ;
// 檔案上傳
void copyFromLocalFile(String local, String path) throws Exception ;
// 拷貝到本地:下載
void copyToLocalFile(String src, String dst) throws Exception ;
// 刪除資料夾
void delete(String path) throws Exception ;
// IO流上傳
void ioUpload(String path, String local) throws Exception ;
// IO流下載
void ioDown(String path, String local) throws Exception ;
// 分塊下載
void blockDown(String path, String local1, String local2) throws Exception ;
}
```
## 2、命令API用法
```java
@Service
public class HdfsFileServiceImpl implements HdfsFileService {
@Resource
private HdfsConfig hdfsConfig ;
@Override
public void mkdirs(String path) throws Exception {
// 1、獲取檔案系統
Configuration configuration = new Configuration();
FileSystem fileSystem = FileSystem.get(new URI(hdfsConfig.getNameNode()),
configuration, "root");
// 2、建立目錄
fileSystem.mkdirs(new Path(path));
// 3、關閉資源
fileSystem.close();
}
@Override
public void isFile(String path) throws Exception {
// 1、獲取檔案系統
Configuration configuration = new Configuration();
FileSystem fileSystem = FileSystem.get(new URI(hdfsConfig.getNameNode()),
configuration, "root");
// 2、判斷檔案和資料夾
FileStatus[] fileStatuses = fileSystem.listStatus(new Path(path));
for (FileStatus fileStatus : fileStatuses) {
if (fileStatus.isFile()) {
System.out.println("檔案:"+fileStatus.getPath().getName());
}else {
System.out.println("資料夾:"+fileStatus.getPath().getName());
}
}
// 3、關閉資源
fileSystem.close();
}
@Override
public void reName(String oldFile, String newFile) throws Exception {
// 1、獲取檔案系統
Configuration configuration = new Configuration();
FileSystem fileSystem = FileSystem.get(new URI(hdfsConfig.getNameNode()),
configuration, "root");
// 2、修改檔名
fileSystem.rename(new Path(oldFile), new Path(newFile));
// 3、關閉資源
fileSystem.close();
}
@Override
public void fileDetail(String path) throws Exception {
// 1、獲取檔案系統
Configuration configuration = new Configuration();
FileSystem fileSystem = FileSystem.get(new URI(hdfsConfig.getNameNode()),
configuration, "root");
// 2、讀取檔案詳情
Remote