C#學習筆記(三):邏輯關系運算符和if語句
阿新 • • 發佈:2019-02-06
同學 判斷 請問 登陸 tasks 不同 入學 根據 重要 條件語句
分支語句和循環語句是程序裏最重要的邏輯。
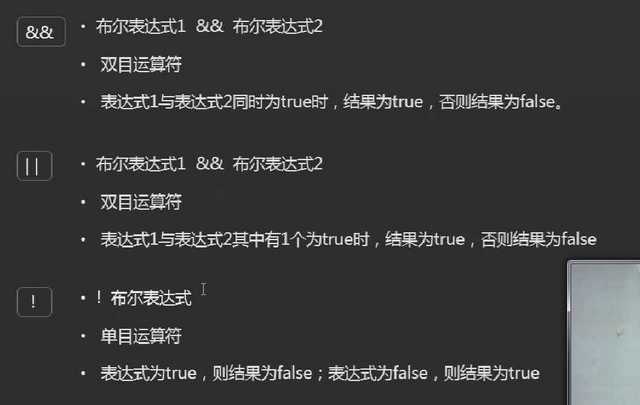
IF語句、分支語句、循環語句
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace w1d4_if { class Program { static void Main(string[] args) { #region if語句 //if語句是C#中處理邏輯分支的一種語句//if關鍵字(布爾表達式){ //} //如果if後的布爾表達式為true則執行關聯塊,否則不執行 #endregion #region if語句練習 //提示內容為大於18歲再能觀看,如果大於18歲,那麽在控制臺輸出“你看到了學習資料” Console.WriteLine("以下內容大於18歲才能觀看"); Console.WriteLine("請問貴君年齡"); int age = int.Parse(Console.ReadLine());if (age > 18) { Console.WriteLine("你看到了學習資料"); } Console.WriteLine("今天你看學習資料花了多少時間(分鐘)"); int time = int.Parse(Console.ReadLine()); if (time > 60) { Console.WriteLine("今天看學習資料花了{0}分鐘時間,看來你很努力哦", time); } Console.WriteLine("請輸入你的年齡"); int age1 = int.Parse(Console.ReadLine()); if (age1 >= 23) { Console.WriteLine("你到了結婚年齡"); } Console.WriteLine("請輸入你的語文成績"); float chinese = float.Parse(Console.ReadLine()); Console.WriteLine("請輸入你的數學成績"); float math = float.Parse(Console.ReadLine()); Console.WriteLine("請輸入你的英語成績"); float english = float.Parse(Console.ReadLine()); bool isConditionA = chinese > 70 && math > 80 && english > 90; bool isConditionB = chinese == 100 && math == 100 && english == 100; bool isConditionC = chinese > 90 && math > 70 || english > 70; if (isConditionA || isConditionB || isConditionC) { Console.WriteLine("非常棒,繼續加油"); } else { Console.WriteLine("繼續努力"); } Console.WriteLine("請輸入用戶名"); string id1 = Console.ReadLine(); Console.WriteLine("請輸入密碼"); string password1 = Console.ReadLine(); string id2 = "admin"; string password2 = "123456"; if (id1 == id2) { if (password1 == password2) { Console.WriteLine("登陸成功"); } } #endregion #region if else語句 //else必須有關聯的條件語句,否則會報錯,錯誤為代碼最前端顯示缺少花括號 //else在所有條件語句不執行時,才執行 Console.WriteLine("請輸入一個整數"); int num = int.Parse(Console.ReadLine()); if (num % 2 == 0) { Console.WriteLine("您輸入的是一個偶數"); } else { Console.WriteLine("您輸入的是一個奇數"); } #endregion #region if else語句練習 Console.WriteLine("請輸入密碼"); string password1 = Console.ReadLine(); string password2 = "888888"; if (password1 == password2) { Console.WriteLine("登陸成功"); } } Console.WriteLine("請輸入一個整數"); int num = int.Parse(Console.ReadLine()); if (num % 2 == 0) { Console.WriteLine("Your input is even"); } else { Console.WriteLine("Your input is odd"); } Console.WriteLine("請輸入你的年齡"); int age = int.Parse(Console.ReadLine()); bool isCondition = ((10 < age && age < 18) || (25 < age && age < 30)); if (isCondition) { Console.WriteLine("可以訪問"); } else { Console.WriteLine("拒絕訪問"); } Console.WriteLine("請輸入第一個整數"); int num1 = int.Parse(Console.ReadLine()); Console.WriteLine("請輸入第二個整數"); int num2 = int.Parse(Console.ReadLine()); Console.WriteLine("請輸入第三個整數"); int num3 = int.Parse(Console.ReadLine()); bool isCondition1 = num1 > num2 && num1 > num3; bool isCondition2 = num2 > num1 && num2 > num3; bool isCondition3 = num3 > num1 && num3 > num1; if (isCondition1) { Console.WriteLine("最大的整數是{0}" , num1); } else if (isCondition2) { Console.WriteLine("最大的整數是{0}", num2); } else if (isCondition3) { Console.WriteLine("最大的整數是{0}", num3); } //要求用戶輸入一個字符,判定這個字符在0-9之間,則輸出您輸入了一個數字,否則輸入的不是數字 while (true) { Console.WriteLine("請輸入一個字符"); char inputChar = char.Parse(Console.ReadLine()); if ((int)inputChar > 48 && (int)inputChar < 57) { Console.WriteLine("您輸入了一個數字"); } else { Console.WriteLine("這不是一個數字"); } Console.WriteLine();//輸入空行 } #endregion #region else if語句 //else if 是一個條件語句 //else if 必須有關聯在其他條件語句後面 //else if 在前一個條件語句不執行的時候執行 //用於配合if及else執行多條件多分支語句 //判定不執行,判定執行,未判定 //else if(布爾表達式){代碼塊} //如果布爾表達式為真則執行代碼塊,否則不執行 if (true) { } else if (true) { } else if (true) { } //根據學生的成績,進行評級 //成績 >= 90 A //成績 >= 80 B //成績 >= 70 C //成績 >= 60 D //成績 < 60 E //出現在前面的條件不能包含後面的條件 //如果包含,出現在else if前面的條件必須小於後面的條件 while(true) { Console.WriteLine("請輸入學生的成績"); int sorce = int.Parse(Console.ReadLine()); if (sorce< 60) { Console.WriteLine("E"); } else if (sorce >= 60) { if (sorce >= 70) { if (sorce >= 80) { if (sorce >= 90) { Console.WriteLine("A"); } else { Console.WriteLine("B"); } } else { Console.WriteLine("C"); } } else { Console.WriteLine("D"); } } } //從三個數中找出最大的數,不考慮相等 Console.WriteLine("請輸入第一個數"); double num1 = double.Parse(Console.ReadLine()); Console.WriteLine("請輸入第二個數"); double num2 = double.Parse(Console.ReadLine()); Console.WriteLine("請輸入第三個數"); double num3 = double.Parse(Console.ReadLine()); bool isCondition1 = num1 > num2 && num1 > num3; bool isCondition2 = num2 > num1 && num2 > num3; bool isCondition3 = num3 > num1 && num3 > num1; if (isCondition1) { Console.WriteLine("最大的數是{0}", num1); } else if (isCondition2) { Console.WriteLine("最大的數是{0}", num2); } else if (isCondition3) { Console.WriteLine("最大的數是{0}", num3); } Console.WriteLine("請輸入密碼"); string password1 = Console.ReadLine(); string password = "888888"; if (password1 == password) { Console.WriteLine("登陸成功"); } else { Console.WriteLine("密碼錯誤,請重新輸入"); string password2 = Console.ReadLine(); if (password2 == password) { Console.WriteLine("登陸成功"); } } string id = "admin"; string password = "888888"; Console.WriteLine("請輸入用戶名"); string id1 = Console.ReadLine(); Console.WriteLine("請輸入密碼"); string password1 = Console.ReadLine(); if (id1 == id) { if (password1 == password) { Console.WriteLine("登陸成功"); } else { Console.WriteLine("密碼錯誤"); } } else { Console.WriteLine("用戶名不存在"); } #endregion } } }
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace 運算符二 { class Program { static void Main(string[] args) { #region 邏輯運算符 //邏輯運算符 //返回是一個布爾值 //參數有不同要求 //&&,||,! //&& //雙目運算符 //兩邊必須是布爾表達式 //表達式均為true的情況,返回true,其他為false //true && true 返回 true //true && false 返回 false //false && true 返回 false //false && false 返回 false //|| //雙目運算符 //兩邊必須是布爾表達式 //表達式一個為true的情況,返回true,其他為false //表達式均為false的情況,返回false,其他為true //true || true 返回 true //true || false 返回 true //false || true 返回 true //false || false 返回 false //! //單目運算符 //右邊必須是布爾表達式 //如果右邊是true,返回false //如果右邊是false,返回true Console.WriteLine(true || true);//true Console.WriteLine(false || true);//true Console.WriteLine(true && true);//true Console.WriteLine(true && false);//false //Console.WriteLine(324 && false);//??,C++允許所有非零整數均為true Console.WriteLine(!true);//false Console.WriteLine(!false);//true #endregion #region 條件運算符 //條件運算符 ?: //三目運算符 //第一表達式?第二表達式:第三表達式 //第一表達式必須是布爾表達式 //第二和第三表達式必須和接收的類型一致 //如果第一表達式為true時,返回第二表達式結果 //如果第一表達式為false時,返回第三表達式結果 Console.WriteLine("請輸入你的血量"); int health = int.Parse(Console.ReadLine()); string message = health > 10 ? "你很安全" : "你有危險"; Console.WriteLine(message); //依次輸入學生的姓名,C#語言的成績,Unity的成績,兩門成績都大於等於90分才能畢業,請輸出最後結果 Console.WriteLine("請輸入你的姓名"); string name = Console.ReadLine(); WriteLine Console.WriteLine("請輸入你的C#語言的成績"); float cSharp = float.Parse(Console.ReadLine()); Console.WriteLine("請輸入你的Unity的成績"); float unity = float.Parse(Console.ReadLine()); string message = (cSharp >= 90 && unity >= 90) ? "可以畢業" : "不能畢業"; Console.WriteLine("{0}同學,你{1}", name, message); //要求用戶輸入一個年份,然後判斷是不是閏年? //閏年判斷 //年份能被400整除(2000) //年份能被4整除,但是不能被100整除(2008) Console.WriteLine("請輸入一個年份"); int year = int.Parse(Console.ReadLine()); bool conditionA = year % 400 == 0; bool conditionB = year % 4 == 0; bool conditionC = year % 100 != 0; string message = (conditionA || (conditionB && conditionC)) ? "是閏年" : "不是閏年"; Console.WriteLine(message); //比較兩個數的大小,求出最大的。 Console.WriteLine("請輸入第一個數字"); float num1 = float.Parse(Console.ReadLine()); Console.WriteLine("請輸入第二個數字"); float num2 = float.Parse(Console.ReadLine()); string message = (num1 > num2) ? num1 + "大" : num2 + "大"; Console.WriteLine(message); //提示用戶輸入一個姓名,然後在控制臺輸出姓名,只要輸入的不是老王,就全是流氓 //1,提取數據 //姓名 //顯示結果 //2,分析數據 //結果 //根據結果,分析條件 //1,老王 姓名 == "老王" //2,流氓 姓名 != "老王" Console.WriteLine("請輸入一個姓名"); string nameTest = Console.ReadLine(); string nameLaoWang = "老王"; string nameLiuMang = "流氓"; string message = (nameTest == nameLaoWang) ? "你是" + nameLaoWang : "你是" + nameLiuMang; Console.WriteLine(message); #endregion } } }
C#學習筆記(三):邏輯關系運算符和if語句