[leetcode] Max Area of Island
阿新 • • 發佈:2018-07-25
style 數組越界 連續 一個 mat exc 搜索 btn bec
Given the above grid, return
分析:題目翻譯一下:要求計算連續1的個數。看題目也是一個DFS問題,當遇到一個1,然後就從上、下、左、又四個方向去搜索。這裏要註意搜索邊界,如果在邊界地方還要搜索,有可能數組越界。 比如
現在加入搜索到第二行第二列的1,然後向上是0,向下是1,再往下還是1,再往下是0。這裏要註意如果是1的話,要用一個visited數組來保存訪問記錄。可以降低重復訪問次數。
代碼如下:
Given a non-empty 2D array grid
of 0‘s and 1‘s, an island is a group of 1
‘s (representing land) connected 4-directionally (horizontal or vertical.) You may assume all four edges of the grid are surrounded by water.
Find the maximum area of an island in the given 2D array. (If there is no island, the maximum area is 0.)
Example 1:
[[0,0,1,0,0,0,0,1,0,0,0,0,0], [0,0,0,0,0,0,0,1,1,1,0,0,0], [0,1,1,0,1,0,0,0,0,0,0,0,0], [0,1,0,0,1,1,0,0,1,0,1,0,0], [0,1,0,0,1,1,0,0,1,1,1,0,0], [0,0,0,0,0,0,0,0,0,0,1,0,0], [0,0,0,0,0,0,0,1,1,1,0,0,0], [0,0,0,0,0,0,0,1,1,0,0,0,0]]Given the above grid, return
6
. Note the answer is not 11, because the island must be connected 4-directionally.
Example 2:
[[0,0,0,0,0,0,0,0]]
0
.
Note: The length of each dimension in the given grid
does not exceed 50.
分析:題目翻譯一下:要求計算連續1的個數。看題目也是一個DFS問題,當遇到一個1,然後就從上、下、左、又四個方向去搜索。這裏要註意搜索邊界,如果在邊界地方還要搜索,有可能數組越界。 比如
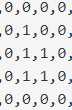
1 publicclass maxareaofisland { 2 int maxarea = 0; 3 public int maxAreaOfIsland(int[][] grid) { 4 boolean[][] visited = new boolean[grid.length][grid[0].length]; 5 for ( int i = 0 ; i < grid.length ; i ++ ){ 6 for ( int j = 0 ; j < grid[i].length ; j ++ ){ 7 int temparea = helper(i,j,grid,visited); 8 maxarea = Math.max(maxarea,temparea); 9 } 10 } 11 return maxarea; 12 } 13 14 private int helper(int i, int j, int[][] grid, boolean[][] visited) { 15 if ( i < 0 || j < 0 || i > grid.length - 1 || j > grid.length - 1) return 0; 16 if ( grid[i][j] == 0 || visited[i][j]) return 0; 17 visited[i][j] = true; 18 return 1 + helper(i+1,j,grid,visited)+helper(i-1,j,grid,visited)+helper(i,j+1,grid,visited)+helper(i,j-1,grid,visited); 19 } 20 }
運行時間23ms,擊敗36.30%的提交。
看了一下其他的方法,基本都是深搜。只是一些技巧問題,就不管了。
[leetcode] Max Area of Island